Description
Given the radius and the position of the center of a circle, implement the function randPoint which generates a uniform random point inside the circle.
Implement the Solution class:
Solution(double radius, double x_center, double y_center) initializes the object with the radius of the circle radius and the position of the center (x_center, y_center).
randPoint() returns a random point inside the circle. A point on the circumference of the circle is considered to be in the circle. The answer is returned as an array [x, y].
Example 1:
Input
[“Solution”, “randPoint”, “randPoint”, “randPoint”]
[[1.0, 0.0, 0.0], [], [], []]
Output
[null, [-0.02493, -0.38077], [0.82314, 0.38945], [0.36572, 0.17248]]
Explanation
Solution solution = new Solution(1.0, 0.0, 0.0);
solution.randPoint(); // return [-0.02493, -0.38077]
solution.randPoint(); // return [0.82314, 0.38945]
solution.randPoint(); // return [0.36572, 0.17248]
Constraints:
0 < radius <= 108
-107 <= x_center, y_center <= 107
At most 3 * 104 calls will be made to randPoint.
Solution
The x-axis and y-axis correspond to uniform pixels, but such numbers will be inside the circle, as shown in the figure below, and may appear in blue.
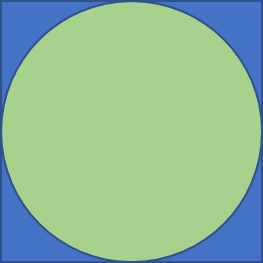
The easiest way to deal with it is to use Pythagorean theorem check whether the random point is in the circle, and if it is not, resample it again until the result is randomly generated.
1 | # O(1) time | O(1) space |
Random Usage
- uniform(): Returns a random float number between two given parameters
- randint(): Returns a random number between the given range
- choice(): Returns a random element from the given sequence
- random(): Returns a random float number between 0 and 1