Description
Given the root of a binary tree, return the leftmost value in the last row of the tree.
Example 1:
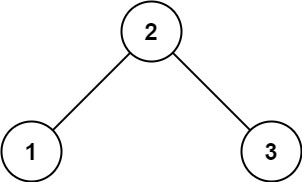
Input: root = [2,1,3]
Output: 1
Example 2:
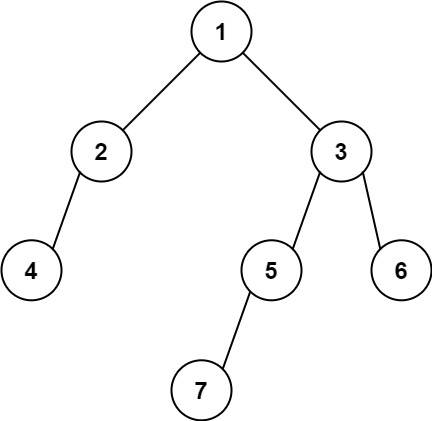
Input: root = [1,2,3,4,null,5,6,null,null,7]
Output: 7
Constraints:
The number of nodes in the tree is in the range [1, 104].
-231 <= Node.val <= 231 - 1
Solution
BFS
- Traverse the tree from left to right, level by level
- Save the first node of each layer
- The answer saved by traversing to the bottom layer is the value in the lower left corner of the tree
1 | # O(nm) time | O(n) space |
- Traverse the tree from right to left, level by level
- The last node traversed is the leftmost value of the tree
1 | # O(n) time | O(n) space |