Description
There is a group of n people labeled from 0 to n - 1 where each person has a different amount of money and a different level of quietness.
You are given an array richer where richer[i] = [ai, bi] indicates that ai has more money than bi and an integer array quiet where quiet[i] is the quietness of the ith person. All the given data in richer are logically correct (i.e., the data will not lead you to a situation where x is richer than y and y is richer than x at the same time).
Return an integer array answer where answer[x] = y if y is the least quiet person (that is, the person y with the smallest value of quiet[y]) among all people who definitely have equal to or more money than the person x.
Example 1:
1 | Input: richer = [[1,0],[2,1],[3,1],[3,7],[4,3],[5,3],[6,3]], quiet = [3,2,5,4,6,1,7,0] |
Example 2:
1 | Input: richer = [], quiet = [0] |
Constraints:
n == quiet.length
1 <= n <= 500
0 <= quiet[i] < n
All the values of quiet are unique.
0 <= richer.length <= n * (n - 1) / 2
0 <= ai, bi < n
ai != bi
All the pairs of richer are unique.
The observations in richer are all logically consistent.
Solution
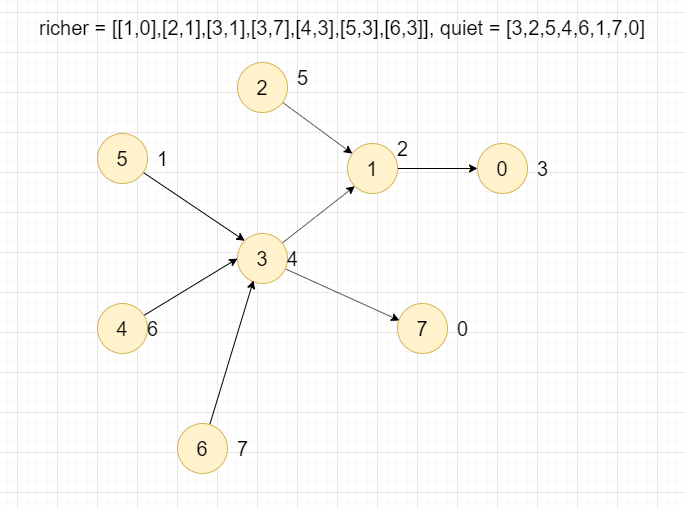
- Draw the graph as required
- Create a mapping table 【connect】:
- key: the vertex of the in-degree
- value: which elements will this vertex of the in-degree point to
- Create an in-degree table 【degree】 :
- key: the value of the vertex;
value: how many in-degree elements there are, for example, vertex 3 has 3 in-degree elements (if the vertex is an index, we only need to create an array/list)
- key: the value of the vertex;
- Find the key/index that are 0 in the in-degree table, that is, 2, 3, 5, 6, and put these values in the queue
- Compare the quiet value of the current popleft value and the quiet value of the outdegree element, and update the element
1 | # O(n+m) time | O(n+m) space |
Gitalking ...