Description
Given the root of a binary tree, return the maximum width of the given tree.
The maximum width of a tree is the maximum width among all levels.
The width of one level is defined as the length between the end-nodes (the leftmost and rightmost non-null nodes), where the null nodes between the end-nodes that would be present in a complete binary tree extending down to that level are also counted into the length calculation.
It is guaranteed that the answer will in the range of a 32-bit signed integer.
Example 1:
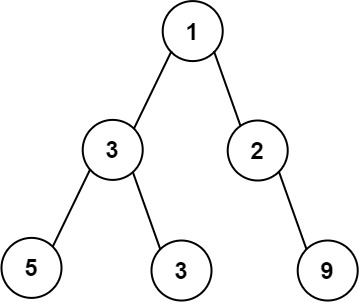
1 | Input: root = [1,3,2,5,3,null,9] |
Example 2:
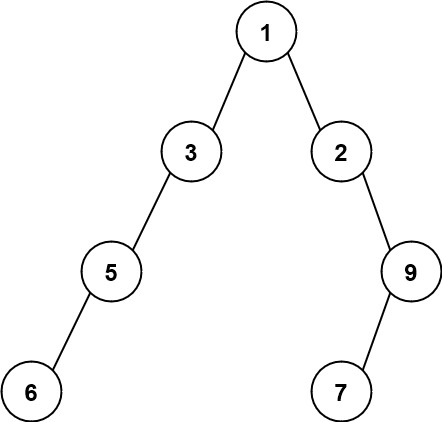
1 | Input: root = [1,3,2,5,null,null,9,6,null,7] |
Example 3:
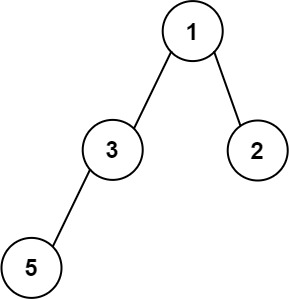
1 | Input: root = [1,3,2,5] |
Constraints:
1 | The number of nodes in the tree is in the range [1, 3000]. |
Soution
Using the full binary tree number, the distance between any two nodes in the same layer (with empty nodes) can be easily calculated, and our BFS returns the layer with the largest distance between the leftmost and the rightmost.
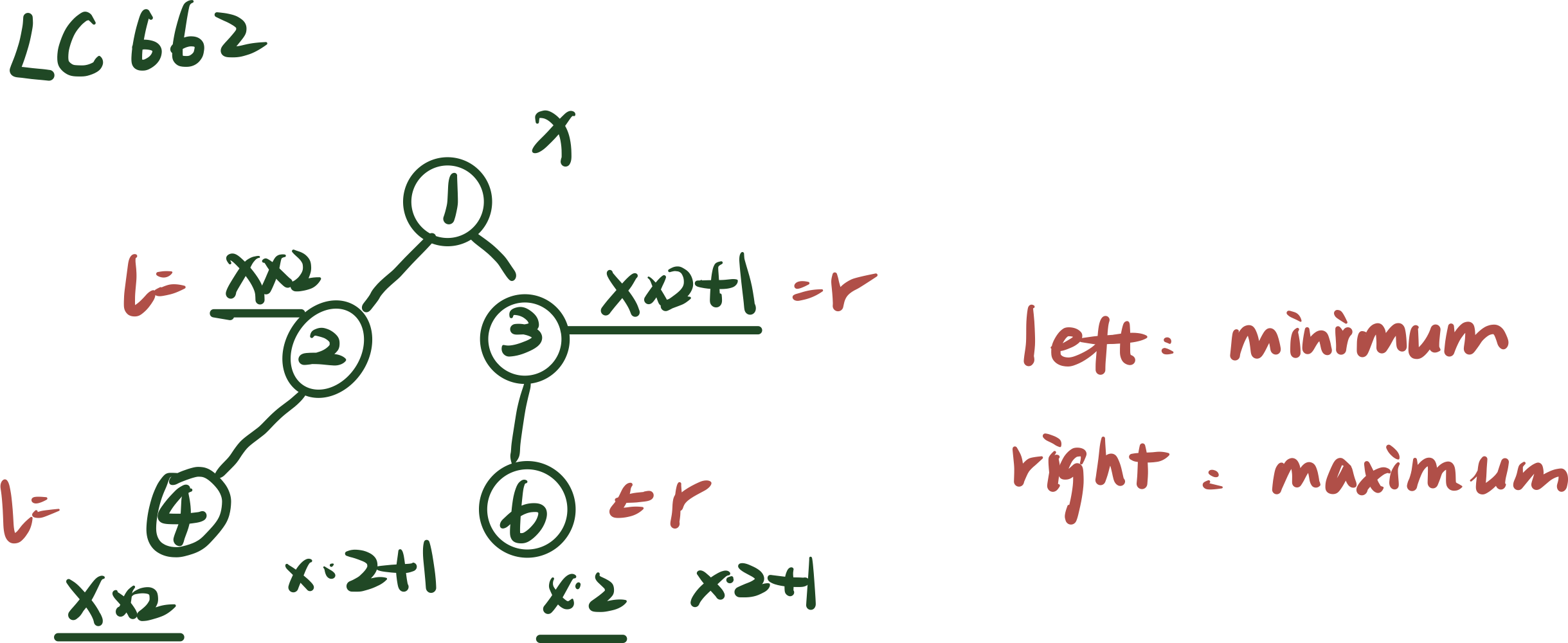
1 | # Definition for a binary tree node. |